Android SDK Setup
Requirements
To use the MessagingSDK, the following is required:
-
A registered Firebase Account
-
Follow the installation steps:
-
Create Android Studio Project
-
Configure FCM in Android Studio Project
-
Messaging.xlm file in value project
-
LocalBroadcastReceiver in Activity project
-
BroadcastReceiver in App project
SDK Installation
Step 1: Open Project in Android Studio IDE
Open Android Studio IDE and open the project that you are working on.
Step 2: Include MessagingSDK Library Dependency
In order to deliver Push Notifications to your App, add the following MessagingSDK dependencies in app.gradle.
build.gradle (Module: app):
...
android {
...
}
dependencies {
...
implementation 'net.mobilengage.sdk:core:0.15.0'
...
}
...
build.gradle:
buildscript {
// ...
dependencies {
// ...
classpath 'com.google.gms:google-services:4.3.1' // google-services plugin
}
}
allprojects {
// ...
repositories {
google()
jcenter()
// ...
}
}
Step 3: Configure FCM in Android Project
You may add the FCM directly using the Android Studio IDE or in Firebase account.
From Android Studio IDE
Open Android Studio IDE and open the project that you are working on
Select your project, go to the Tools tab, and select the Firebase option:
- Select Cloud Messaging, and then click to Set up Firebase Cloud Messaging.
- Connect your app to an existing Firebase project or create a new Firebase project in the console.
- Add FCM to the Application.
- Once you have connected the Android Studio project to a Firebase Project and Added CFM to the App, you shall see the following confirmation screen:
NOTE
From the Android Studio IDE configuration, do not complete the "Handle message" step as the SDK will do it for you.
Or you can configure the project using firebase console directly.
By console:
- Go to Firebase console
-
You can create a new project or select an existing one.
-
You must append the package of the Android project in the configuration of the firebase project and configure the options for sending push notifications.
- Add Firebase into the project.
- Download the google-service.json file and incorporate it into the project.
- Incorporate our SDK into the project and get started.
Step 4: Obtain and Add Messaging.xml file in values project.
Obtain the configuration file Messaging.xml from the Mobile Messaging Platform. This is found in the Preferences section under the Push tab after completing the initial App Integration setup.
Example of Messaging.xml file:
<string name="messaging_host" translatable="false" templateMergeStrategy="preserve">https://elastic.messangi.me/aemon/v2</string>
<string name="messaging_app_token" translatable="false">YourAppToken</string>
<bool name="analytics_allowed">true or false</bool>
<bool name="location_allowed">true or false</bool>
<bool name="logging_allowed">true or false</bool>
<bool name="locationPermissionAtStartup">true or false</bool>
</resources>
Put the configuration file in the values folder of the Android project.
Once you have completed these steps, Push Notifications will be able to be delivered to the Device managed by the OS functionality.
Self Implementation
The SDK handles incoming push notifications automatically by default, but there is the possibility to customize the handling of notifications using the following class in the project:
Create class in App project named CustomMessagingService:
import android.util.Log;
import com.google.firebase.messaging.RemoteMessage;
import com.messaging.sdk.MessagingFirebaseService;
import com.messaging.sdk.MessagingNotification;
public class CustomMessagingService extends MessagingFirebaseService {
....
@Override
public void onNewToken(String s) {
super.onNewToken(s);
}
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
//example to custom
MessagingNotification messagingNotification = new MessagingNotification(remoteMessage);
messaging = Messaging.getInstance(this);
messaging.sendGlobalEventToActivity(Messaging.ACTION_GET_NOTIFICATION,messagingNotification);
}
}
It is important to override onNewToken method to use super.onNewToken(s) (heredity) for the proper functionality of the SDK.
For this custom class you can receive messages overwriting the method onMessageReceived.
Remember if you want to use CustomMessagingService, declare service in the Manifest.xml of the App project:
<application
.....
<activity
</activity>
<service
android:name=".CustomMessagingService"
android:permission="com.google.android.c2dm.permission.SEND">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
<action android:name="com.google.android.c2dm.intent.RECEIVE" />
</intent-filter>
</service>
</application>
</manifest>
To handle push notifications (automatically or custom), a BroadcastReceiver must be implemented in the project with the characteristics shown below:
...
import …...
public class MessagingNotificationReceiver extends BroadcastReceiver{
...
...
@Override
public void onReceive(Context context, Intent intent) {
boolean hasError=intent.getBooleanExtra(Messaging.INTENT_EXTRA_HAS_ERROR,true);
if (!hasError ) {
String action=intent.getAction();
Serializable data = intent.getSerializableExtra(Messaging.INTENT_EXTRA_DATA);
if(intent.getAction().equals(Messaging.ACTION_GET_NOTIFICATION)&& data!=null){
........
}
private void sendEventToActivity(String action,Serializable something, Context context) {
if(something!=null) {
Intent intent = new Intent(action);
intent.putExtra(Messaging.INTENT_EXTRA_DATA, something);
intent.putExtra(Messaging.INTENT_EXTRA_HAS_ERROR, something == null);
LocalBroadcastManager.getInstance(context).sendBroadcast(intent);
}
}
To handle push notifications with the app in foreground, you can use the sendEventToActivity method in the global BroadcastReceiver in order to send the notification information to the activity that requires it, for this it will also be necessary to implement a LocalBroadcastReceiver in the aforementioned activity.
To implement the LocalBroadcastReceiver please see the User, Device and Notification Management section.
Now for the handling of push notifications automatically by the SDK just by implementing the BroadcastReceiver shown above, in the project it is enough.
Notification Management
For the management of users, devices and the handling of push notifications in the foreground, the following steps must be implemented:
Step 1: Put LocalBroadcastReceiver in Activity project
...
import com.messaging.sdk.Messaging;
public class MainActivity extends AppCompatActivity{
...
...
@Override
protected void onStart() {
super.onStart();
LocalBroadcastManager.getInstance(this).registerReceiver(mReceiver,
new IntentFilter(Messaging.ACTION_FETCH_DEVICE));
.....
}
The Receiver of the LocalBroadcastManager instances must be registered
using the corresponding Intentfilter, example: Messaging.ACTION_FETCH_DEVICE.
Then you can create a method:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Messaging messaging=Messaging.getInstance(this);
dothisForRequestUser();
}
//get Instance of Messaging..
public void dothisForRequestUser() {
Messaging.fetchDevice(false);
}
Using fetchDevice device delivers the instance of a device by internal memory, local memory or a service.
You can also request devices or you can handle push notifications with the app in foreground.
Finally in OnDestroy cycle live of Activity:
@Override
protected void onDestroy() {
LocalBroadcastManager.getInstance(this).unregisterReceiver(mReceiver);
super.onDestroy();
}
This method let unregister receivers when activity finishes.
For get a response from fetch device request (example used), or user request or handle notification push , you must implement a LocalBroadcastReceiver in the activity:
private BroadcastReceiver mReceiver=new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
boolean hasError=intent.getBooleanExtra(Messaging.INTENT_EXTRA_HAS_ERROR,true);
if (!hasError ) {
Serializable data=intent.getSerializableExtra(Messaging.INTENT_EXTRA_DATA);
if(data == null){
if(progressBar.isShown()){
progressBar.setVisibility(View.GONE);
}
return;
}
switch (intent.getAction()){
case Messaging.ACTION_REGISTER_DEVICE:
messagingDevice = (MessagingDevice) data;
....
break;
case Messaging.ACTION_FETCH_DEVICE:
messagingDevice = (MessagingDevice) data;
if(messagingUser == null){
Messaging.fetchUser(getApplicationContext(),false);
}
....
break;
case Messaging.ACTION_SAVE_DEVICE:
messagingDevice = (MessagingDevice) data;
if(messagingUser != null){
Messaging.fetchUser(getApplicationContext(),true);
}
...
break;
case Messaging.ACTION_FETCH_USER:
messagingUser = (MessagingUser) data;
...
break;
case Messaging.ACTION_SAVE_USER:
messagingUser = (MessagingUser) data;
...
break;
case Messaging.ACTION_GET_NOTIFICATION:
messagingNotification = (MessagingNotification) data;
....
break;
case Messaging.ACTION_GET_NOTIFICATION_OPENED:
messagingNotification = (MessagingNotification) data;
...
break;
case Messaging.ACTION_FETCH_LOCATION:
MessagingLocation messagingLocation = (MessagingLocation) data;
break;
default:
break;
}
}else{
Toast.makeText(getApplicationContext(),"An error occurred on action "
+intent.getAction(),Toast.LENGTH_LONG).show();
}
......
}
};
//you can see all the implementation in example app
In case of handling push notifications in background and foreground, a global BroadcastReceiver must be implemented in the project.
Step 2: Put BroadcastReceiver in app project
...
import …...
public class MessagingNotificationReceiver extends BroadcastReceiver{
...
...
@Override
public void onReceive(Context context, Intent intent) {
boolean hasError=intent.getBooleanExtra(Messaging.INTENT_EXTRA_HAS_ERROR,true);
if (!hasError ) {
String action=intent.getAction();
Serializable data = intent.getSerializableExtra(Messaging.INTENT_EXTRA_DATA);
//optional code to determinate if app is Background or not
ActivityManager.RunningAppProcessInfo myProcess = new ActivityManager.RunningAppProcessInfo();
ActivityManager.getMyMemoryState(myProcess);
boolean isInBackground = myProcess.importance != ActivityManager.RunningAppProcessInfo.IMPORTANCE_FOREGROUND;
if(intent.getAction().equals(Messaging.ACTION_GET_NOTIFICATION)&& data!=null){
...
}else if(intent.getAction().equals(Messaging.ACTION_FETCH_LOCATION)){
wayLatitude = intent.getDoubleExtra(Messaging.INTENT_EXTRA_DATA_lAT,0.00);
wayLongitude = intent.getDoubleExtra(Messaging.INTENT_EXTRA_DATA_lONG,0.00);
Location location=new Location(LOCATION_SERVICE);
location.setLatitude(wayLatitude);
location.setLongitude(wayLongitude);
MessagingLocation messagingLocation=new MessagingLocation(location);
if(isInBackground){
...
}else{
sendEventToActivity(Messaging.ACTION_FETCH_LOCATION,messagingLocation,context);
...
}
}else if(intent.getAction().equals(Messaging.ACTION_GEOFENCE_ENTER)
||intent.getAction().equals(Messaging.ACTION_GEOFENCE_EXIT)
||intent.getAction().equals(Messaging.ACTION_FETCH_GEOFENCE)){
messagingCircularRegions=(ArrayList<MessagingCircularRegion>)data;
if(isInBackground){
...
}else{
sendEventToActivity(Messaging.ACTION_FETCH_GEOFENCE,messagingCircularRegions,context);
...
}
}else{
Toast.makeText(context,alertMessage,Toast.LENGTH_LONG).show();
}
//to send data to Activity if app is foreground
private void sendEventToActivity(String action,Serializable something, Context context) {
if(something!=null) {
Intent intent = new Intent(action);
intent.putExtra(Messaging.INTENT_EXTRA_DATA, something);
intent.putExtra(Messaging.INTENT_EXTRA_HAS_ERROR, something == null);
LocalBroadcastManager.getInstance(context).sendBroadcast(intent);
}else{
//error
}
}
It is important to use, please declare BroadcastReceiver in Manifest.xml of app project, example:
<application
.....
<activity
</activity>
<receiver
android:name=".MessagingNotificationReceiver"
android:enabled="true"
android:permission="${applicationId}.permission.pushReceive"
android:exported="false"
tools:ignore="Instantiatable">
<intent-filter>
<action android:name="com.messaging.sdk.PUSH_NOTIFICATION" />
<action android:name="com.messaging.sdk.ACTION_FETCH_LOCATION" />
<action android:name="com.messaging.sdk.ACTION_GEOFENCE_ENTER" />
<action android:name="com.messaging.sdk.ACTION_GEOFENCE_EXIT" />
</intent-filter>
</receiver>
</application>
<permission
android:name="${applicationId}.permission.pushReceive"
android:protectionLevel="signature" />
<uses-permission android:name="${applicationId}.permission.pushReceive" />
</manifest>
Step 3: Receive Notifications in Background
To handle push notifications in background or foreground, this code must be implemented in the activity intended to display the content of the push notification.
//for handle notification from background
Bundle extras = null;
if(Static.extras!=null){
...
extras=Static.extras;
Static.extras=null;
}else{
extras=getIntent().getExtras();
...
}
if(extras!=null){
isBackground=extras.getBoolean("isInBackground",false);
if(isBackground) {
Serializable data = extras.getSerializable(Messaging.INTENT_EXTRA_DATA);
messagingNotification=(MessagingNotification)data;
.....
}else {
//to process notification from background mode
MessagingNotification notification=Messaging.checkNotification(extras);
.......
}
}
The extra parameters and isBackground were sent by the global BroadcastReceiver when a push notification arrives.
Usage
To make use of the functionalities that the MessagingSDK offers, utilize the Messaging class.
To obtain the instance of this class:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Messaging messaging=Messaging.getInstance(this);
}
//get Instance of Messaging..
All the services offered by this library are provided by means of an instance of the Messaging class, and it can be obtained that it was indicated later.
Obtain User Data
Method to obtain each User by Device that is registered from the service.
Whenforsecallservice=true, search device parameters through the service.
@Override
protected void onResume() {
super.onResume();
Messaging.fetchUser(getApplicationContext(), true);
}
you can use in the activity:
messagingUser.addProperty(key,value);
For asing property to the user, example of a Property: Juan, Alvarado, [email protected] or 555-55-55, and example of key : example name, LastName or Phone.
then for update User data, you can use:
messagingUser.save(getApplicationContext());;
This method usesLocalBroadcastReceiver to send Instance from the SDK to Activity. (Please refer to Step 1).
Obtain Device
Receive the OS version of the user’s device, example:
@Override
protected void onStart() {
super.onStart();
LocalBroadcastManager.getInstance(this).registerReceiver(mReceiver,
new IntentFilter(Messaging.ACTION_FETCH_DEVICE));
}
...
@Override
protected void onResume() {
super.onResume();
Messaging.fetchDevice(false);
}
private BroadcastReceiver mReceiver=new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
boolean hasError=intent.getBooleanExtra(Messaging.INTENT_EXTRA_HAS_ERROR,true);
if (!hasError ) {
Serializable data=intent.getSerializableExtra(Messaging.INTENT_EXTRA_DATA);
if(intent.getAction().equals(Messaging.ACTION_FETCH_DEVICE)&& data!=null){
messagingDevice = (MessagingDevice) data; //you can cast this for get information
.....
}else if(intent.getAction().equals(Messaging.ACTION_FETCH_USER)&& data!=null){
messagingUser =(MessagingUser) data;
.......
}else{
Toast.makeText(getApplicationContext(),"An error occurred on action "
+intent.getAction(),Toast.LENGTH_LONG).show();
}
......
}
};
//you can see all the implementation in example app
@Override
protected void onDestroy() {
LocalBroadcastManager.getInstance(this).unregisterReceiver(mReceiver);
}
MessagingDevice is used to handle Device parameters in the SDK.
To obtain this device instance, the SDK can provide it in three ways:
-
local memory
-
internal memory
-
service
To add a Tag to a Device, you may Save and it will immediately be updated in the database.
messagingDev.addTagToDevice(tags);
This method usesBroadcastReceiver to send Instance from the SDK to Activity. (Please refer to Step 1).
messagingDevice.save(getApplicationContext());
Deep Linking
MessagingSDK allows for Push Notifications to contain a link to a section inside of the Application or to a Web Page link.
For this we have 3 modalities:
-
Payload
-
URL Schema
-Universal Links
Payload
The "click_action" property sent through the Push Notification will be used, which has two cases:
-
Notification in Foreground: handled by the SDK.
-
Notification in Background: handled by the OS.
Notification in Foreground
The Notification will be handled by the SDK taking the property "click_action", which should have a value as shown in the example:
"click_action": "com.ogangi.Messangi.SDK.Demo.ExampleActivity "
This is then processed by the SDK, allowing the host app to create an icon in the notification tray that when the user opens the activity it executes the defined action.
The declaration of the activity must be done in the AndroidManifest.xml file:
<activity android: name = ".ExampleActivity">
<intent-filter>
<action android: name = "com.ogangi.Messangi.SDK.Demo.ExampleActivity" />
<category android: name = "android.intent.category.DEFAULT" />
</intent-filter>
</activity>
In MainActivity of the Demo App, a Foreground Notification is sent:
Arrival of the Push Notification to the App that shows the detail of the Notification and in the upper part, it displays the generation of the Notification due to the Click-Action parameter. To generate the notification you can see the launchNotification method in MainActivity of the Demo app.
Then open the notification palette:
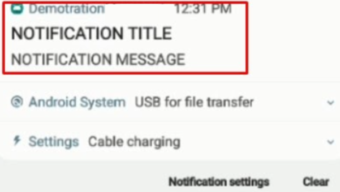
When the Notification is clicked and is opened, the predetermined activity is executed and data is obtained with the following structure:
Bundle extras=getIntent().getExtras();
boolean enable=extras.getBoolean("enable",false);
additionalData = new HashMap<>();
if(extras!=null && !enable) {
for (String key : extras.keySet()) {
additionalData.put(key, extras.getString(key));
messangiData.add(key + " , " + extras.getString(key));
}
.....
}else{
Serializable data = extras.getSerializable(Messaging.INTENT_EXTRA_DATA);
messagingNotification=(MessagingNotification)data;
additionalData=messagingNotification.getAdditionalData();
if(additionalData!=null && additionalData.size()>0) {
messangiData.add("Title: " + messagingNotification.getTitle());
messangiData.add("Body: " + messagingNotification.getBody());
messangiData.add("ClickAction: " + messagingNotification.getClickAction());
messangiData.add("DeepUriLink: " + messagingNotification.getDeepUriLink());
for (Map.Entry entry : messagingNotification.getAdditionalData().entrySet()) {
if (!entry.getKey().equals("profile")) {
messangiData.add(entry.getKey() + " , " + entry.getValue());
}
}
}
}
.....
Finally the screen of the open activity is presented as a product of the parameter "Click_Action"
"click_action":" com.ogangi.Messangi.SDK.Demo.ExampleActivity
Notification in Background
In this case, the Notification will be handled by the OS with its native behavior.
The activity when the Notification is opened by the user must be declared, it is important to remember that the Notification must contain the field:
"click_action ":"com.ogangi.Messangi.SDK.Demo.ExampleActivity "
Then open the notification palette:
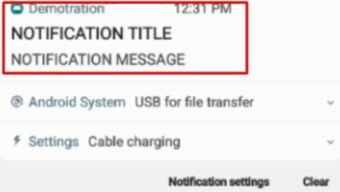
Finally the screen of the open activity is presented as a product of the parameter "Click_Action".
This action was performed using the OS native behavior, but it is important that the Notification contains the "click_action" field:
"com.ogangi.Messangi.SDK.Demo.ExampleActivity"
The obtained data can also be processed using the following code in one class, example ExampleActivity.class:
Bundle extras=getIntent().getExtras();
boolean enable=extras.getBoolean("enable",false);
additionalData = new HashMap<>();
if(extras!=null && !enable) {
for (String key : extras.keySet()) {
additionalData.put(key, extras.getString(key));
messangiData.add(key + " , " + extras.getString(key));
}
.....
}else{
Serializable data = extras.getSerializable(Messaging.INTENT_EXTRA_DATA);
messagingNotification=(MessagingNotification)data;
additionalData=messagingNotification.getAdditionalData();
if(additionalData!=null&& additionalData.size()>0) {
messangiData.add("Title: " + messagingNotification.getTitle());
messangiData.add("Body: " + messagingNotification.getBody());
messangiData.add("ClickAction: " + messagingNotification.getClickAction());
messangiData.add("DeepUriLink: " + messagingNotification.getDeepUriLink());
for (Map.Entry entry : messagingNotification.getAdditionalData().entrySet()) {
if (!entry.getKey().equals("profile")) {
messangiData.add(entry.getKey() + " , " + entry.getValue());
}
}
}
}
Url Schema
The Notification will be handled by the SDK taking the property "link", which should have a value as shown in the example:
"link": "exampleapp://example/example?param1=1"
This is then processed by the SDK, allowing the host app to open the activity and take the data that brings the push notification.
The declaration of the activity must be done in the AndroidManifest.xml file:
<activity android: name = ".ExampleURLSchemasActivity">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data
android:host="example"
android:pathPrefix="/example"
android:scheme="exampleapp" />
</intent-filter>
</activity>
The "link" property sent through the Push Notification will be used, which has two cases:
-
Notification in Foreground: handled by the SDK.
-
Notification in Background: handled by the OS.
Handle notification in Foreground
In MainActivity of the Demo App, a Foreground Notification is sent:
To open the Activity you can see the launchBrowser method in MainActivity of the Demo app.
After the push notification is sent, the activity ExampleURLSchemasActivity (example in demo app) will open automatically:
Handle Notification in Background
In this case, the Notification will be handled by the OS with its native behavior.
The activity when the Notification is opened by the user must be declared, it is important to remember that the Notification must contain the field:
"link":"exampleapp://example/example?param1=1"
Then open the notification palette:
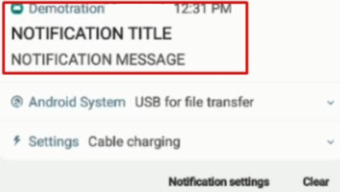
After clicking on the notification, the following Activity will open:
The obtained data can also be processed using the following code in (Example Activity create in app demo), ExampleURLSchemasActivity.class:
Bundle extras=getIntent().getExtras();
boolean enable=extras.getBoolean("enable",false);
additionalData = new HashMap<>();
if(extras!=null && !enable) {
for (String key : extras.keySet()) {
additionalData.put(key, extras.getString(key));
messangiData.add(key + " , " + extras.getString(key));
}
.....
}else{
Serializable data = extras.getSerializable(Messaging.INTENT_EXTRA_DATA);
messagingNotification=(MessagingNotification)data;
additionalData=messagingNotification.getAdditionalData();
if(additionalData!=null&& additionalData.size()>0) {
messangiData.add("Title: " + messagingNotification.getTitle());
messangiData.add("Body: " + messagingNotification.getBody());
messangiData.add("ClickAction: " + messagingNotification.getClickAction());
messangiData.add("DeepUriLink: " + messagingNotification.getDeepUriLink());
for (Map.Entry entry : messagingNotification.getAdditionalData().entrySet()) {
if (!entry.getKey().equals("profile")) {
messangiData.add(entry.getKey() + " , " + entry.getValue());
}
}
}
}
Universal Link
The Notification will be handled by the SDK taking the property "link", which should have a value as shown in the example:
"link": "
This is then processed by the SDK, allowing the host app to open the activity and take the data that brings the push notification.
The declaration of the activity must be done in the AndroidManifest.xml file:
<activity android: name = ".ExampleUrlActivity">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:host="www.plantplaces.com" android:pathPrefix="/colorcapture.shtml" android:scheme="http" />
</intent-filter>
</activity>
The "link" property sent through the Push Notification will be used, which has two cases:
-
Notification in Foreground: handled by the SDK.
-
Notification in Background: handled by the OS.
Notification in Foreground
In MainActivity of the Demo App, a Foreground Notification is sent:
To open the Activity you can see the launchBrowser method in MainActivity of the Demo app.
After the push notification is sent, the app demo will open automatically by selection,
the the activity (example using in demo app) ExampleUrlActivity,class will open:
The obtained data can also be processed using the following code in ExampleURLActivity.class:
Bundle extras=getIntent().getExtras();
boolean enable=extras.getBoolean("enable",false);
additionalData = new HashMap<>();
if(extras!=null && !enable) {
for (String key : extras.keySet()) {
additionalData.put(key, extras.getString(key));
messangiData.add(key + " , " + extras.getString(key));
}
.....
}else{
Serializable data = extras.getSerializable(Messaging.INTENT_EXTRA_DATA);
messagingNotification=(MessagingNotification)data;
additionalData=messagingNotification.getAdditionalData();
if(additionalData!=null&& additionalData.size()>0) {
messangiData.add("Title: " + messagingNotification.getTitle());
messangiData.add("Body: " + messagingNotification.getBody());
messangiData.add("ClickAction: " + messagingNotification.getClickAction());
messangiData.add("DeepUriLink: " + messagingNotification.getDeepUriLink());
for (Map.Entry entry : messagingNotification.getAdditionalData().entrySet()) {
if (!entry.getKey().equals("profile")) {
messangiData.add(entry.getKey() + " , " + entry.getValue());
}
}
}
}
Notification handle in Background
In this case, the Notification will be handled by the OS with its native behavior.
The activity when the Notification is opened by the user must be declared, it is important to remember that the Notification must contain the field:
"link":"
When a push notification arrives, open the notification palette:
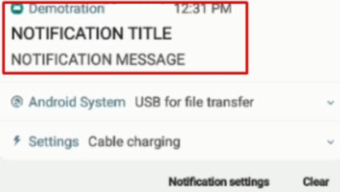
After clicking on the notification, the following Activity will open:
The obtained data can also be processed using the following code in ExampleURLActivity.class:
Bundle extras=getIntent().getExtras();
boolean enable=extras.getBoolean("enable",false);
additionalData = new HashMap<>();
if(extras!=null && !enable) {
for (String key : extras.keySet()) {
additionalData.put(key, extras.getString(key));
messangiData.add(key + " , " + extras.getString(key));
}
.....
}else{
Serializable data = extras.getSerializable(Messaging.INTENT_EXTRA_DATA);
messagingNotification=(MessagingNotification)data;
additionalData=messagingNotification.getAdditionalData();
if(additionalData!=null&& additionalData.size()>0) {
messangiData.add("Title: " + messagingNotification.getTitle());
messangiData.add("Body: " + messagingNotification.getBody());
messangiData.add("ClickAction: " + messagingNotification.getClickAction());
messangiData.add("DeepUriLink: " + messagingNotification.getDeepUriLink());
for (Map.Entry entry : messagingNotification.getAdditionalData().entrySet()) {
if (!entry.getKey().equals("profile")) {
messangiData.add(entry.getKey() + " , " + entry.getValue());
}
}
}
}
Updated over 2 years ago